mirror of
https://github.com/thegeeklab/wp-opentofu.git
synced 2024-09-20 01:42:45 +02:00
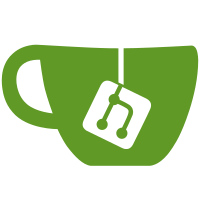
Terraform will pull and cache config. This causes issues if you try to change remotes as the cache will be pushed to your remote when it initializes the new remote.
126 lines
2.4 KiB
Go
126 lines
2.4 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"os/exec"
|
|
"strings"
|
|
"io/ioutil"
|
|
|
|
"github.com/drone/drone-plugin-go/plugin"
|
|
)
|
|
|
|
type terraform struct {
|
|
Remote remote `json:"remote"`
|
|
Plan bool `json:"plan"`
|
|
Vars map[string]string `json:"vars"`
|
|
Cacert string `json:"ca_cert"`
|
|
Sensitive bool `json:"sensitive"`
|
|
}
|
|
|
|
type remote struct {
|
|
Backend string `json:"backend"`
|
|
Config map[string]string `json:"config"`
|
|
}
|
|
|
|
func main() {
|
|
|
|
workspace := plugin.Workspace{}
|
|
vargs := terraform{}
|
|
|
|
plugin.Param("workspace", &workspace)
|
|
plugin.Param("vargs", &vargs)
|
|
plugin.MustParse()
|
|
|
|
var commands []*exec.Cmd
|
|
remote := vargs.Remote
|
|
if vargs.Cacert != "" {
|
|
commands = append(commands, installCaCert(vargs.Cacert))
|
|
}
|
|
if remote.Backend != "" {
|
|
commands = append(commands, deleteCache())
|
|
commands = append(commands, remoteConfigCommand(remote))
|
|
}
|
|
commands = append(commands, planCommand(vargs.Vars))
|
|
if !vargs.Plan {
|
|
commands = append(commands, applyCommand())
|
|
}
|
|
commands = append(commands, deleteCache())
|
|
|
|
for _, c := range commands {
|
|
c.Env = os.Environ()
|
|
c.Dir = workspace.Path
|
|
c.Stdout = os.Stdout
|
|
c.Stderr = os.Stderr
|
|
if !vargs.Sensitive {
|
|
trace(c)
|
|
}
|
|
|
|
err := c.Run()
|
|
if err != nil {
|
|
fmt.Println("Error!")
|
|
fmt.Println(err)
|
|
os.Exit(1)
|
|
}
|
|
fmt.Println("Command completed successfully")
|
|
}
|
|
|
|
}
|
|
|
|
func installCaCert(cacert string) *exec.Cmd {
|
|
ioutil.WriteFile("/usr/local/share/ca-certificates/ca_cert.crt", []byte(cacert), 0644)
|
|
return exec.Command(
|
|
"update-ca-certificates",
|
|
)
|
|
}
|
|
|
|
func deleteCache() *exec.Cmd {
|
|
return exec.Command(
|
|
"rm",
|
|
"-rf",
|
|
".terraform",
|
|
)
|
|
}
|
|
|
|
func remoteConfigCommand(config remote) *exec.Cmd {
|
|
args := []string{
|
|
"remote",
|
|
"config",
|
|
fmt.Sprintf("-backend=%s", config.Backend),
|
|
}
|
|
for k, v := range config.Config {
|
|
args = append(args, fmt.Sprintf("-backend-config=%s=%s", k, v))
|
|
}
|
|
return exec.Command(
|
|
"terraform",
|
|
args...,
|
|
)
|
|
}
|
|
|
|
func planCommand(variables map[string]string) *exec.Cmd {
|
|
args := []string{
|
|
"plan",
|
|
"-out=plan.tfout",
|
|
}
|
|
for k, v := range variables {
|
|
args = append(args, "-var")
|
|
args = append(args, fmt.Sprintf("%s=%s", k, v))
|
|
}
|
|
return exec.Command(
|
|
"terraform",
|
|
args...,
|
|
)
|
|
}
|
|
|
|
func applyCommand() *exec.Cmd {
|
|
return exec.Command(
|
|
"terraform",
|
|
"apply",
|
|
"plan.tfout",
|
|
)
|
|
}
|
|
|
|
func trace(cmd *exec.Cmd) {
|
|
fmt.Println("$", strings.Join(cmd.Args, " "))
|
|
}
|